check repository here
Comets
☄️ Comets: Animating Particles in Swift
animation made by kevin as part of Voicy design
implements Bennet van der Linden medium Comets: Animating Particles in Swift
Example
// Customize your comet
let width = view.bounds.width
let height = view.bounds.height
let comets = [Comet(startPoint: CGPoint(x: 100, y: 0),
endPoint: CGPoint(x: 0, y: 100),
lineColor: UIColor.white.withAlphaComponent(0.2),
cometColor: UIColor.white),
Comet(startPoint: CGPoint(x: 0.4 * width, y: 0),
endPoint: CGPoint(x: width, y: 0.8 * width),
lineColor: UIColor.white.withAlphaComponent(0.2),
cometColor: UIColor.white),
Comet(startPoint: CGPoint(x: 0.8 * width, y: 0),
endPoint: CGPoint(x: width, y: 0.2 * width),
lineColor: UIColor.white.withAlphaComponent(0.2),
cometColor: UIColor.white),
Comet(startPoint: CGPoint(x: width, y: 0.2 * height),
endPoint: CGPoint(x: 0, y: 0.25 * height),
lineColor: UIColor.white.withAlphaComponent(0.2),
cometColor: UIColor.white),
Comet(startPoint: CGPoint(x: 0, y: height - 0.8 * width),
endPoint: CGPoint(x: 0.6 * width, y: height),
lineColor: UIColor.white.withAlphaComponent(0.2),
cometColor: UIColor.white),
Comet(startPoint: CGPoint(x: width - 100, y: height),
endPoint: CGPoint(x: width, y: height - 100),
lineColor: UIColor.white.withAlphaComponent(0.2),
cometColor: UIColor.white),
Comet(startPoint: CGPoint(x: 0, y: 0.8 * height),
endPoint: CGPoint(x: width, y: 0.75 * height),
lineColor: UIColor.white.withAlphaComponent(0.2),
cometColor: UIColor.white)]
// draw line track and animate
for comet in comets {
view.layer.addSublayer(comet.drawLine())
view.layer.addSublayer(comet.animate())
}
Requirements
Comets is written in Xcode 10, Swift 5.0, iOS 9.0 Required
📲 Installation
Comets is available through Cocoapods or Carthage.
Cocoapods
pod "Comets"
Carthage
github "cruisediary/Comets" ~> 0.3.1
❤️ Contribution
Pull requests are always welcomed 🏄🏼
👨💻 Author
cruz, [email protected]
🛡 License
Comets is available under the MIT license. See the LICENSE file for more info.
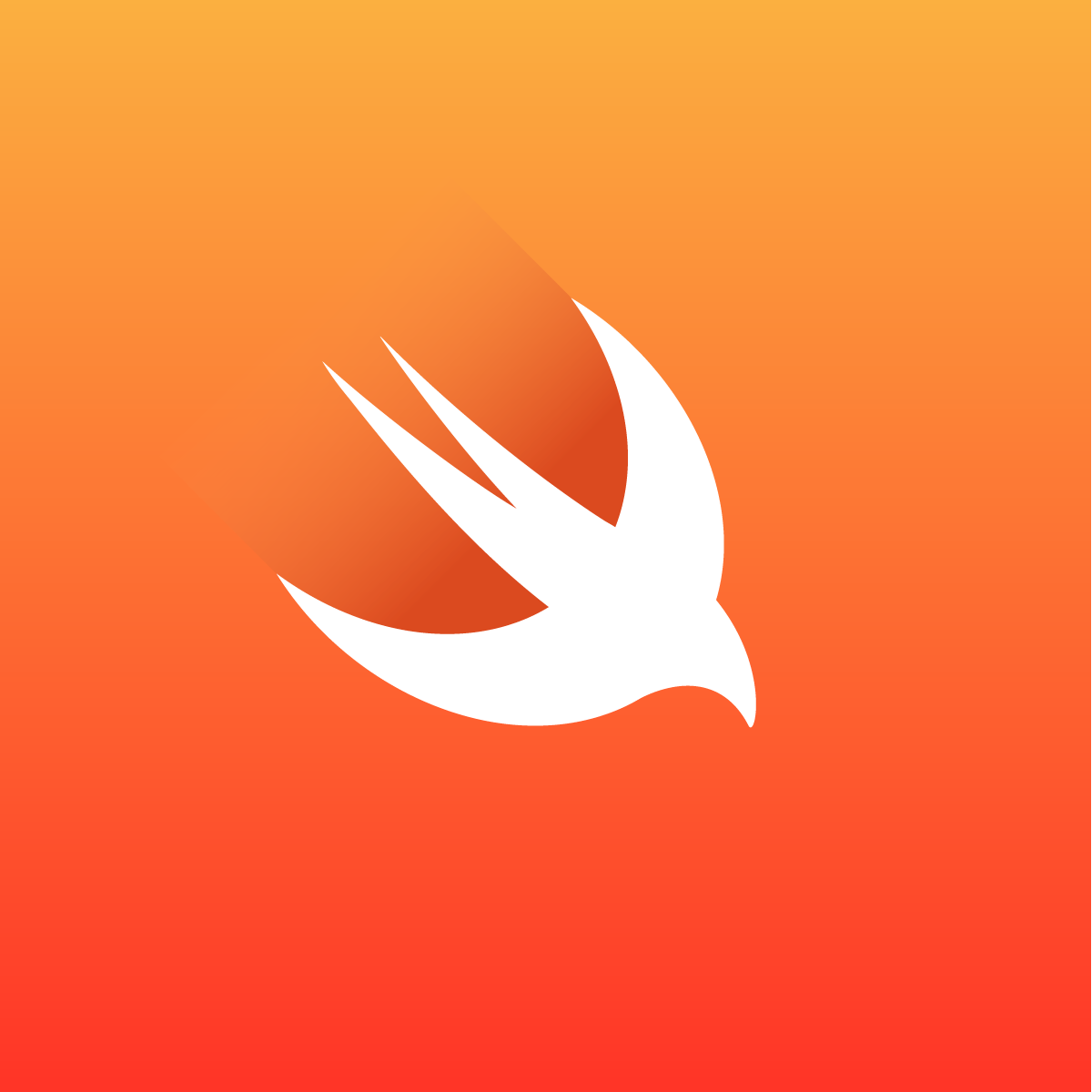
Swift
Swift is Apple’s programming language for developing iOS, macOS, and watchOS applications. It’s fast, safe, and easy to learn, with features like type safety and memory management, enabling efficient app development.
GitHub - hirohisa/PageController: Infinite paging controller, scrolling through contents and title bar scrolls with a delay
GitHub - Quick/Nimble: A Matcher Framework for Swift and Objective-C
GitHub - roberthein/BouncyLayout: Make. It. Bounce.
GitHub - fjcaetano/RxWebSocket: Reactive WebSockets
GitHub - Kitura/Kitura: A Swift web framework and HTTP server.
GitHub - exyte/ProgressIndicatorView: An iOS progress indicator view library written in SwiftUI
GitHub - rolandleth/LTHRadioButton: A radio button with a pretty animation
GitHub - ashishkakkad8/AKSwiftSlideMenu: Slide Menu (Drawer) in Swift
GitHub - BastiaanJansen/toast-swift: Customizable Swift Toast view built with UIKit. 🍞
GitHub - Jintin/Swimat: An Xcode formatter plug-in to format your swift code.
GitHub - thoughtbot/Runes: Infix operators for monadic functions in Swift
GitHub - tid-kijyun/Kanna: Kanna(鉋) is an XML/HTML parser for Swift.
GitHub - ParkGwangBeom/Windless: Windless makes it easy to implement invisible layout loading view.
GitHub - pkluz/PKHUD: A Swift based reimplementation of the Apple HUD (Volume, Ringer, Rotation,…) for iOS 8.
GitHub - shtnkgm/ImageTransition: Library for smooth animation of images during transitions.
GitHub - hyperoslo/Sugar: :coffee: Something sweet that goes great with your Cocoa
GitHub - mukeshthawani/TriLabelView: A triangle shaped corner label view for iOS written in Swift.
GitHub - yonat/BatteryView: Simple battery shaped UIView
GitHub - suzuki-0000/CountdownLabel: Simple countdown UILabel with morphing animation, and some useful function.
GitHub - sindresorhus/DockProgress: Show progress in your app's Dock icon
GitHub - Yalantis/GuillotineMenu: Our Guillotine Menu Transitioning Animation implemented in Swift reminds a bit of a notorious killing machine.
GitHub - MrSkwiggs/Netswift: A type-safe, high-level networking solution for Swift apps
GitHub - evermeer/AttributedTextView: Easiest way to create an attributed UITextView (with support for multiple links and from html)
GitHub - rosberry/texstyle: Format iOS attributed strings easily
GitHub - ra1028/DiffableDataSources: 💾 A library for backporting UITableView/UICollectionViewDiffableDataSource.
GitHub - Mijick/PopupView: Popups presentation made simple (SwiftUI)
GitHub - envoy/Embassy: Super lightweight async HTTP server library in pure Swift runs in iOS / MacOS / Linux
GitHub - shima11/FlexiblePageControl: A flexible UIPageControl like Instagram.
GitHub - ChiliLabs/CHIOTPField: CHIOTPField is a set of textfields that can be used for One-time passwords, SMS codes, PIN codes, etc. Mady by @ChiliLabs - https://chililabs.io
GitHub - LeonardoCardoso/SectionedSlider: iOS 11 Control Center Slider
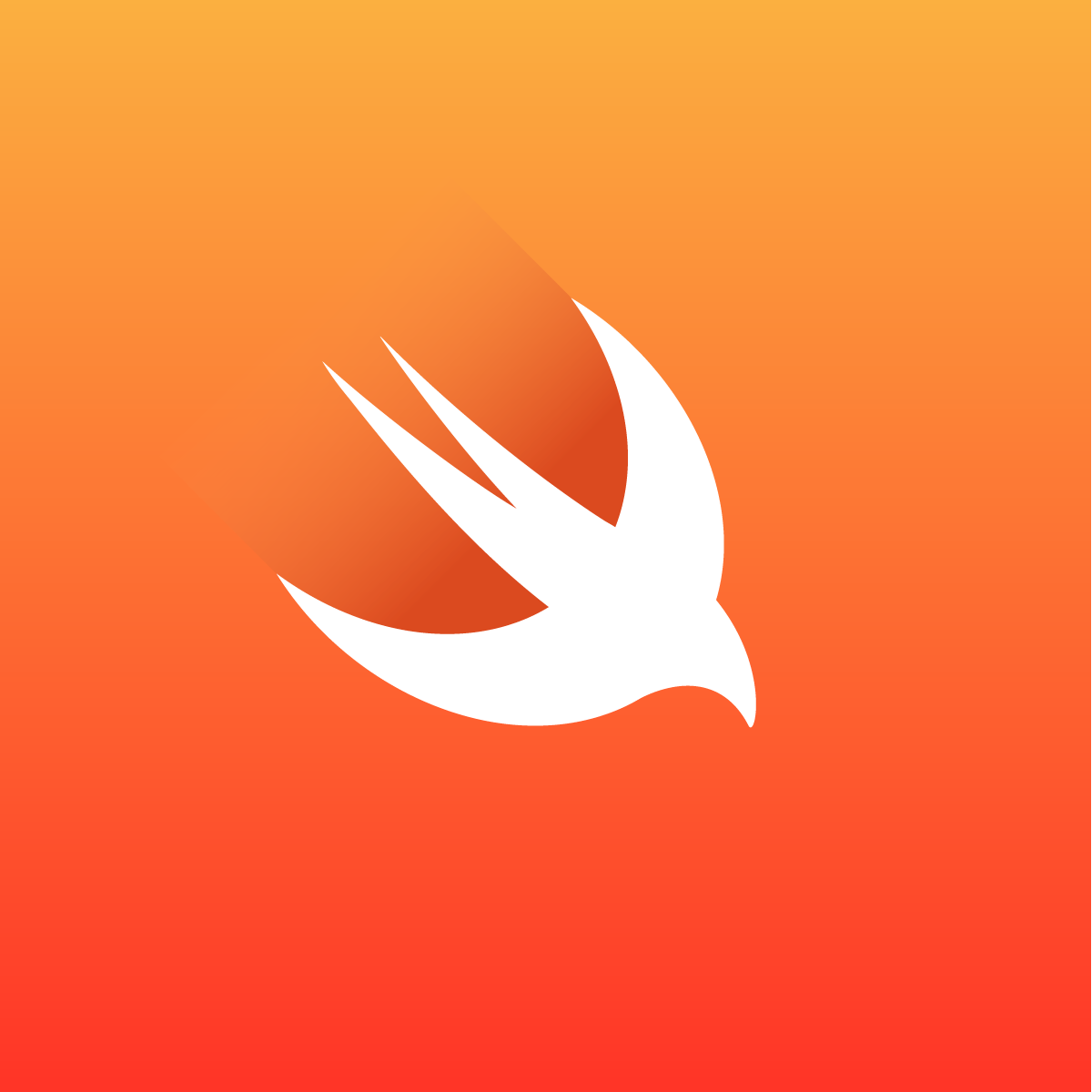
More on Swift
Programming Tips & Tricks
Code smarter, not harder—insider tips and tricks for developers.
Using Lua's Garbage Collector for Fine-Tuned Memory Management in Large Applications
#1
Leveraging Lua's Environment for Dynamic Code Execution and Security
#2
Mastering Lua's Table Manipulation: Advanced Techniques for Handling Large Data Sets
#3
Enhancing Code Readability and Debugging with Lua's Debug Library
#4
Leveraging Lua's Coroutines for Efficient Concurrency and Asynchronous Programming
#5
Leveraging Lua's Tail Call Optimization for Efficient Recursion and Avoiding Stack Overflow in Deep Recursive Functions
#6
Creating Custom Iterators in Lua for Traversing Complex Data Structures Like Graphs or Trees
#7
How to Maximize the Speed of Data Lookup in Lua Using Hash Tables and Optimized Table Management
#8
Advanced Techniques for Asynchronous Programming in Lua Using Coroutines for High-Performance Systems
#9
Mastering Efficient Memory Management in Lua with Weak Tables for Optimal Resource Utilization
#10
Error Solutions
Turn frustration into progress—fix errors faster than ever.
Visual Studio Crashes When Opening a File or Debugging a Project
#1
Visual Studio Cannot Connect to GitHub or Other Version Control System
#2
Visual Studio Freezes During Build or Debug Process
#3
Visual Studio Fails to Build Project with "Unable to Start Program" Error
#4
Visual Studio Shows "Cannot Open the Solution" Error Message When Opening a Project
#5
Visual Studio Crashes on Startup with "The application has encountered an error" Message
#6
Visual Studio Fails to Detect Changes in Files When Using Git Integration
#7
Visual Studio Crashes with "Unknown Exception" When Trying to Build Solution
#8
Visual Studio Hangs During Debugging with "Unable to Start Program" Error
#9
Visual Studio Fails to Load Solution with "The system cannot find the file specified" Error
#10
Shortcuts
The art of speed—shortcuts to supercharge your workflow.
Maximize Your Code Navigation with Cmd + Option + Left Arrow!
#1
Feel Like a Pro with Cmd + Control + D: Quickly View Definitions Like Never Before!
#2
Unlock Speed and Precision with Cmd + Shift + M: Maximize Your Productivity!
#3
Don’t Panic, Use Cmd + Option + I to Open the Developer Tools Now!
#4
Stop Wasting Time and Jump to Your File in an Instant with Cmd + Shift + O!
#5
Quickly Fix Your Code with ‘Cmd + Option + L’ for Instant Code Formatting!
#6
Transform Your Workflow with ‘Cmd + Shift + N’ to Open a New Window in No Time!
#7
Say Goodbye to Mouse: Master ‘Cmd + Shift + F’ to Search Your Entire Codebase!
#8
Struggling to Find Files? Hit ‘Cmd + P’ and Jump Right to Your File!
#9
Never Lose Your Progress Again: Use ‘Cmd + Z’ to Undo Mistakes Instantly!
#10
Made with ❤️
to provide resources in various ares.