check repository here
@abao_ai_bot in Telegram and Discord
- Leetcode Solutions in Rust
- AdventOfCode Solutions in Rust
- Google Solutions in Rust
This project demonstrates how to create Data Structures and to implement Algorithms using programming language Rust All the solutions here are crafted with love and their performance beats 99% of other solutions on the leetcode website. To speed up your learning with generative AI, visit abao.ai and create a FREE account to manage your telegram @username. Add @abao_ai_bot to your telegram group with your friends to learn rust coding together. You can ask GPT, Gemini, Claude coding questions with your friends and tutors in the group together. Other Image and Video generation models are also available for paid subscribers.
Data Structures
- Stack & Queue ( Vec, VecDeque )
- Linked List ( Option<Box
> ) - Hash Tables ( HashMap, HashSet )
- Tree Tables ( BTreeMap, BTreeSet )
- Binary Search Tree ( Option<Rc<RefCell
>> ) - Binary Heaps & Priority Queue ( BinaryHeap )
- Graphs ( Vec<Vec
> ) - Union Find ( UnionFind )
- Trie ( Trie )
Algorithms
- Bit Manipulation & Numbers
- Stability in Sorting
- Heapsort
- Binary Search
- Kth Smallest Elements
- Permutations
- Subsets
- BFS Graph
- DFS Graph
- Dijkstra’s Algorithm
- Tree Traversals
- BFS
- DFS
- in-order
- pre-order
- post-order
- Topological Sort
- Detect cycle in an undirected graph
- Detect a cycle in a directed graph
- Count connected components in a graph
- Find strongly connected components in a graph
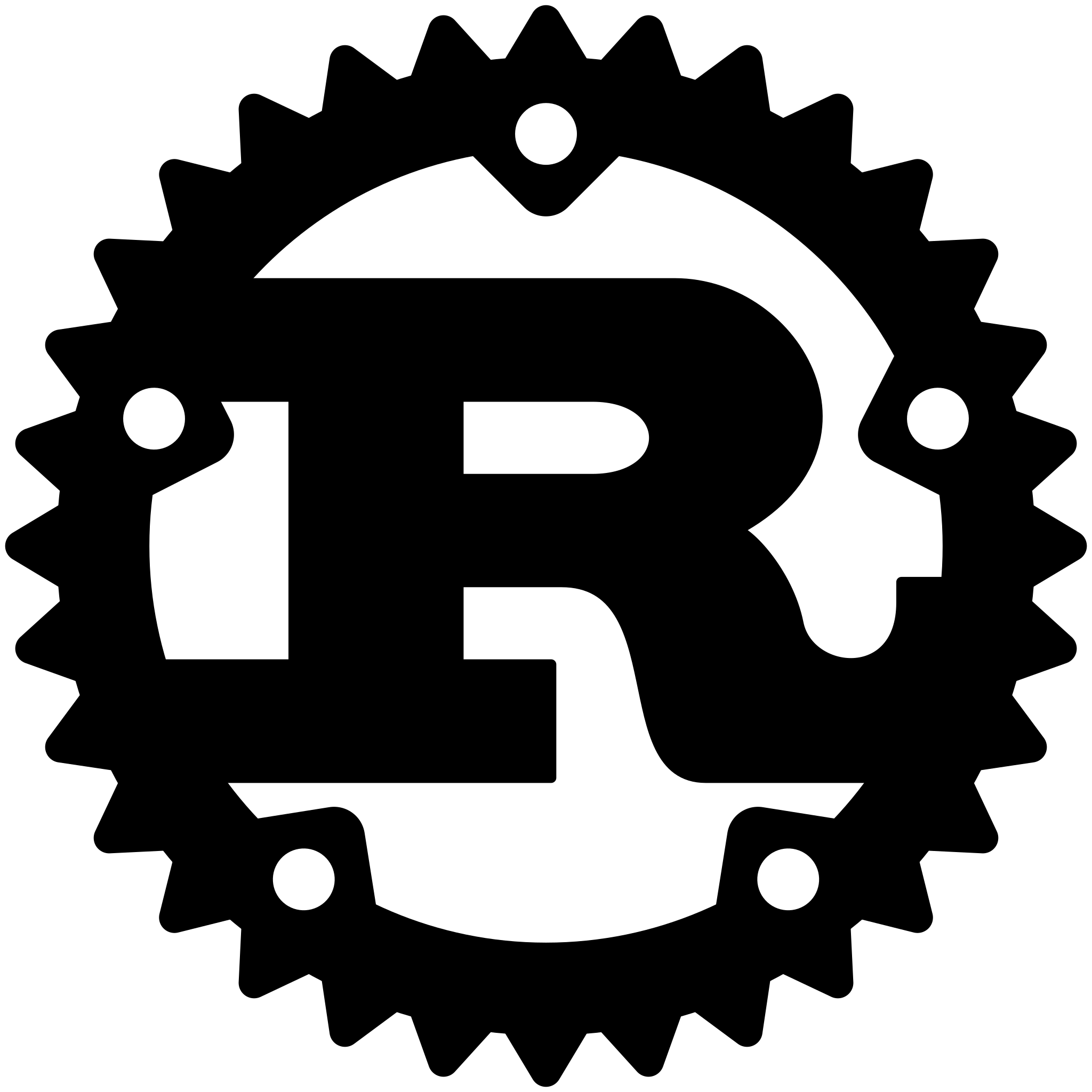
Rust
Rust is a modern programming language focused on safety, speed, and concurrency. It prevents common bugs like null pointer dereferencing and data races, making it ideal for system programming and high-performance applications.
Create business apps like assembling blocks | ILLA Cloud
GitHub - 0x59616e/SteinsOS: An operating system written in Rust
GitHub - j0ru/kickoff: Minimalistic program launcher
Workflow runs · rust-lang/rustup
GitHub - paradigmxyz/artemis: A simple, modular, and fast framework for writing MEV bots in Rust.
Workflow runs · sigp/lighthouse
GitHub - flox/flox: Developer environments you can take with you
Unified Architecture - OPC Foundation
MaidSafe
ttyperacer / terminal-typeracer · GitLab
GitHub - cloudhead/rx: 👾 Modern and minimalist pixel editor
GitHub - rust-ethereum/ethabi: Encode and decode smart contract invocations
GitHub - watchexec/watchexec: Executes commands in response to file modifications
GitHub - osa1/tiny: A terminal IRC client
GitHub - sergree/whatbpm: 💓 Today's Trending Values for EDM Production
GitHub - rsaarelm/magog: A roguelike game in Rust
GitHub - autonomys/subspace: Subspace Network reference implementation
GitHub - nicohman/eidolon: Provides a single TUI-based registry for drm-free, wine and steam games on linux, accessed through a rofi launch menu.
GitHub - shshemi/tabiew: A lightweight, terminal-based application to view and query delimiter separated value formatted documents, such as CSV or TSV files.
GitHub - joamag/boytacean: A GB emulator that is written in Rust 🦀!
GitHub - chaosprint/glicol: Graph-oriented live coding language and music/audio DSP library written in Rust
GitHub - wasmerio/winterjs: Winter is coming... ❄️
GitHub - withoutboats/notty: A new kind of terminal
GitHub - cfal/shoes: A multi-protocol proxy server written in Rust (HTTP, HTTPS, SOCKS5, Vmess, Vless, Shadowsocks, Trojan, Snell)
GitHub - eigerco/beerus: A stateless trustless Starknet light client in Rust 🦀
GitHub - quilt/etk: evm toolkit
GitHub - pop-os/system76-power: System76 Power Management
GitHub - Limeth/ethaddrgen: Custom Ethereum vanity address generator made in Rust
The Tor Project / Core / Arti · GitLab
Production
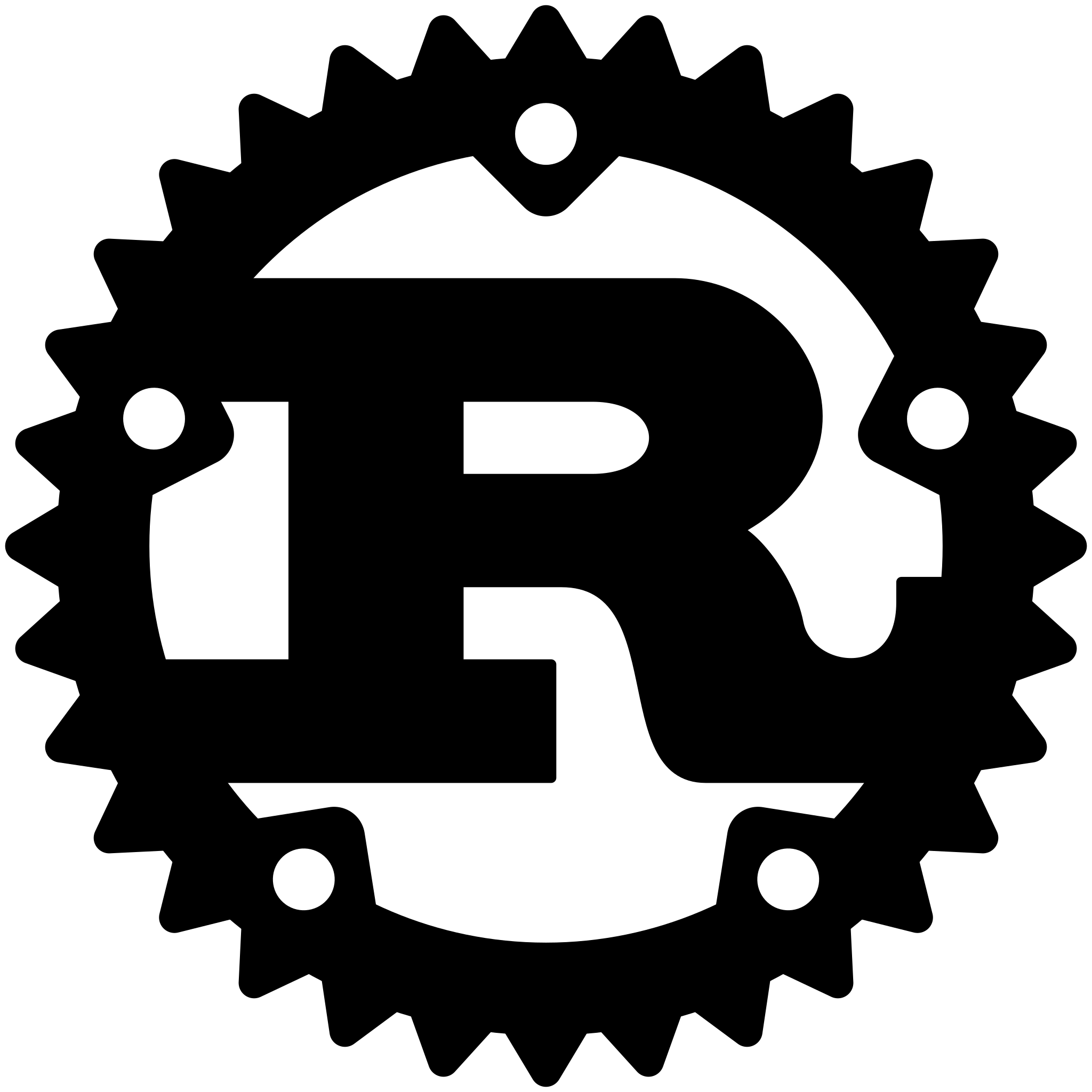
More on Rust
Programming Tips & Tricks
Code smarter, not harder—insider tips and tricks for developers.
Using Lua's Garbage Collector for Fine-Tuned Memory Management in Large Applications
#1
Leveraging Lua's Environment for Dynamic Code Execution and Security
#2
Mastering Lua's Table Manipulation: Advanced Techniques for Handling Large Data Sets
#3
Enhancing Code Readability and Debugging with Lua's Debug Library
#4
Leveraging Lua's Coroutines for Efficient Concurrency and Asynchronous Programming
#5
Leveraging Lua's Tail Call Optimization for Efficient Recursion and Avoiding Stack Overflow in Deep Recursive Functions
#6
Creating Custom Iterators in Lua for Traversing Complex Data Structures Like Graphs or Trees
#7
How to Maximize the Speed of Data Lookup in Lua Using Hash Tables and Optimized Table Management
#8
Advanced Techniques for Asynchronous Programming in Lua Using Coroutines for High-Performance Systems
#9
Mastering Efficient Memory Management in Lua with Weak Tables for Optimal Resource Utilization
#10
Error Solutions
Turn frustration into progress—fix errors faster than ever.
Visual Studio Crashes When Opening a File or Debugging a Project
#1
Visual Studio Cannot Connect to GitHub or Other Version Control System
#2
Visual Studio Freezes During Build or Debug Process
#3
Visual Studio Fails to Build Project with "Unable to Start Program" Error
#4
Visual Studio Shows "Cannot Open the Solution" Error Message When Opening a Project
#5
Visual Studio Crashes on Startup with "The application has encountered an error" Message
#6
Visual Studio Fails to Detect Changes in Files When Using Git Integration
#7
Visual Studio Crashes with "Unknown Exception" When Trying to Build Solution
#8
Visual Studio Hangs During Debugging with "Unable to Start Program" Error
#9
Visual Studio Fails to Load Solution with "The system cannot find the file specified" Error
#10
Shortcuts
The art of speed—shortcuts to supercharge your workflow.
Maximize Your Code Navigation with Cmd + Option + Left Arrow!
#1
Feel Like a Pro with Cmd + Control + D: Quickly View Definitions Like Never Before!
#2
Unlock Speed and Precision with Cmd + Shift + M: Maximize Your Productivity!
#3
Don’t Panic, Use Cmd + Option + I to Open the Developer Tools Now!
#4
Stop Wasting Time and Jump to Your File in an Instant with Cmd + Shift + O!
#5
Quickly Fix Your Code with ‘Cmd + Option + L’ for Instant Code Formatting!
#6
Transform Your Workflow with ‘Cmd + Shift + N’ to Open a New Window in No Time!
#7
Say Goodbye to Mouse: Master ‘Cmd + Shift + F’ to Search Your Entire Codebase!
#8
Struggling to Find Files? Hit ‘Cmd + P’ and Jump Right to Your File!
#9
Never Lose Your Progress Again: Use ‘Cmd + Z’ to Undo Mistakes Instantly!
#10
Made with ❤️
to provide resources in various ares.